as a replacement for existing Java services at Semantico.
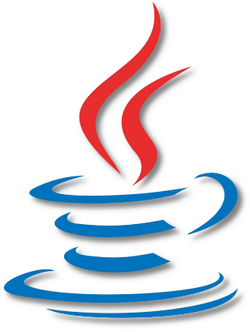
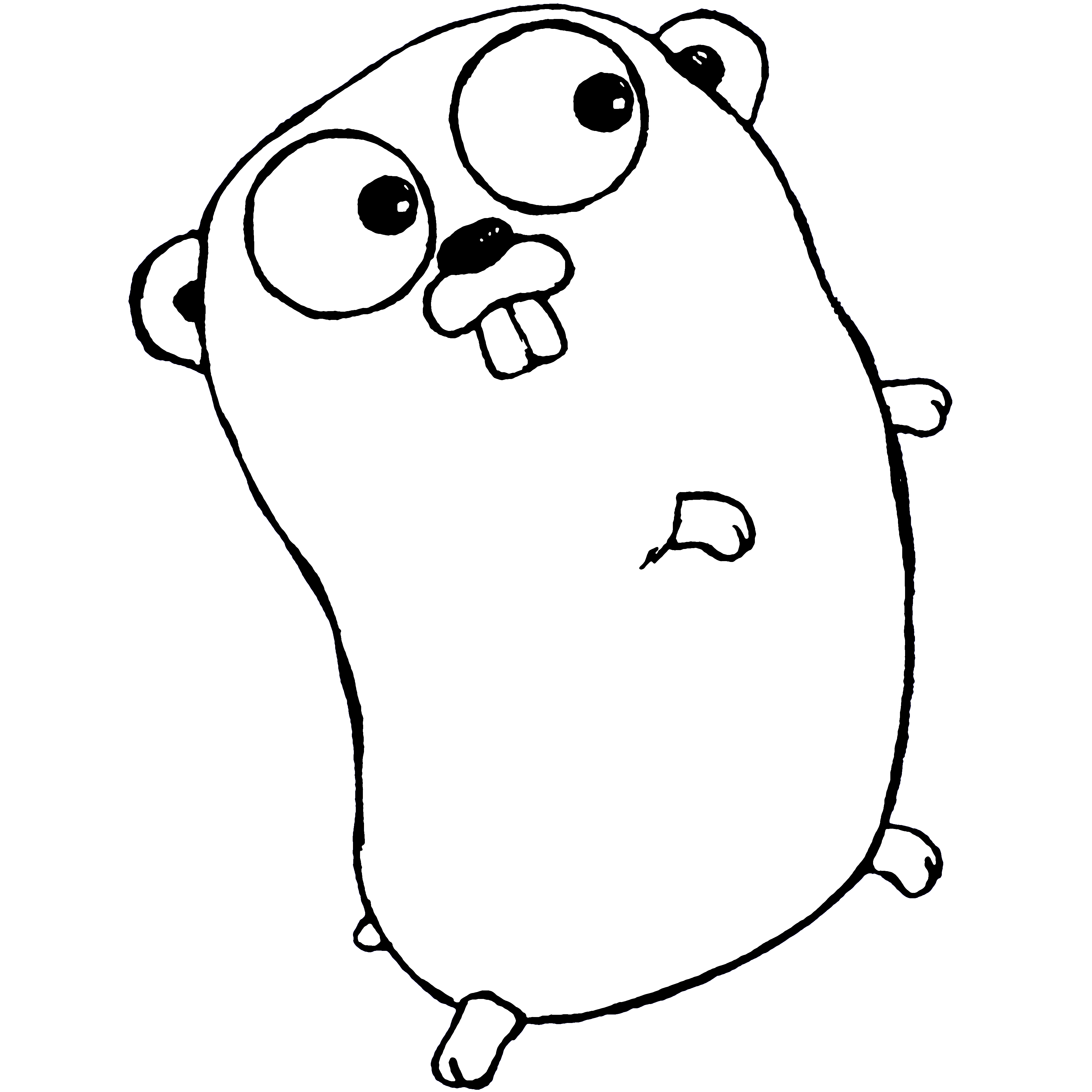
- "Java Web Developer" for Semantico
- Mostly develop in XSLT and XQuery
- Have recently been piloting a new role as a maintenance developer
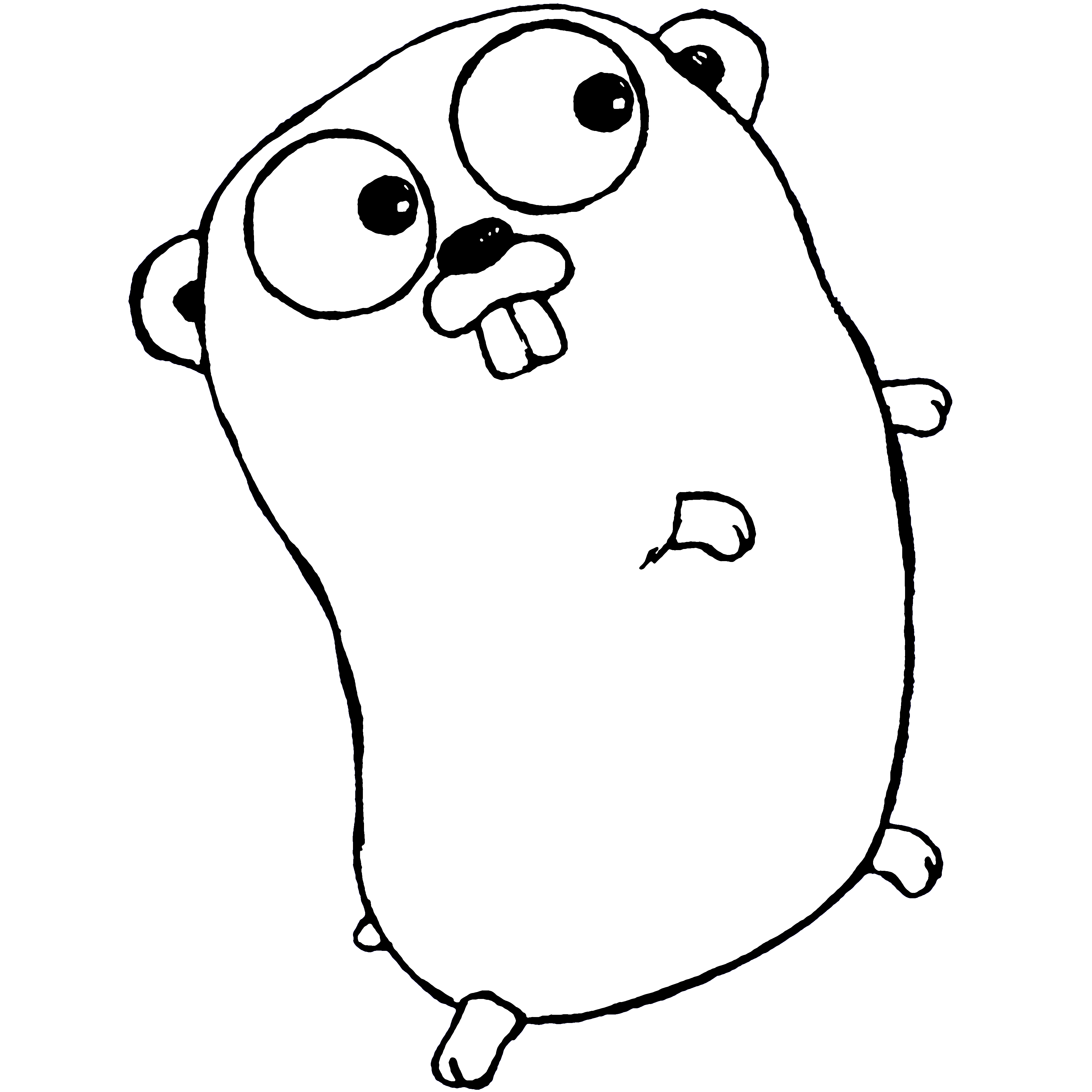
"Its concurrency mechanisms make it easy to write programs that
get the most out of multicore and networked machines,
while its novel type system enables flexible and modular program construction."
void Bar() {}
}
func (f *Foo) Bar() {}
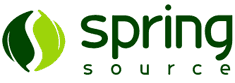
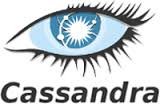
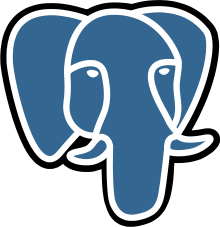
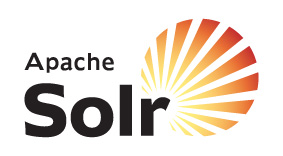
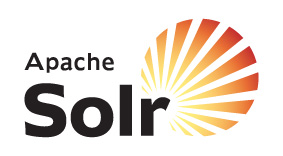
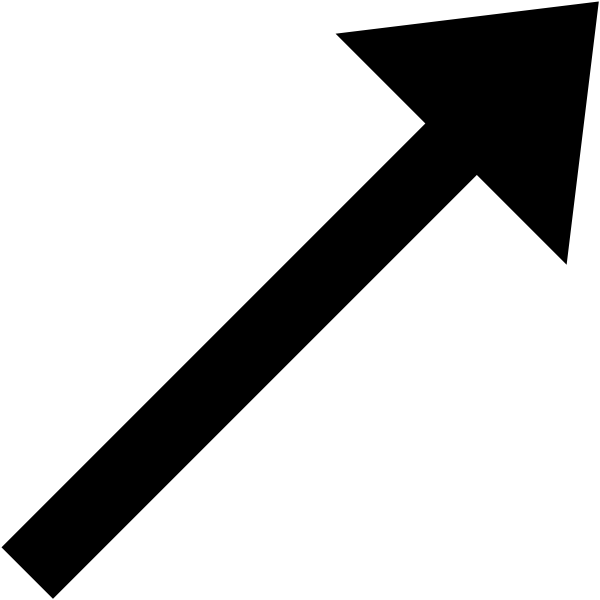
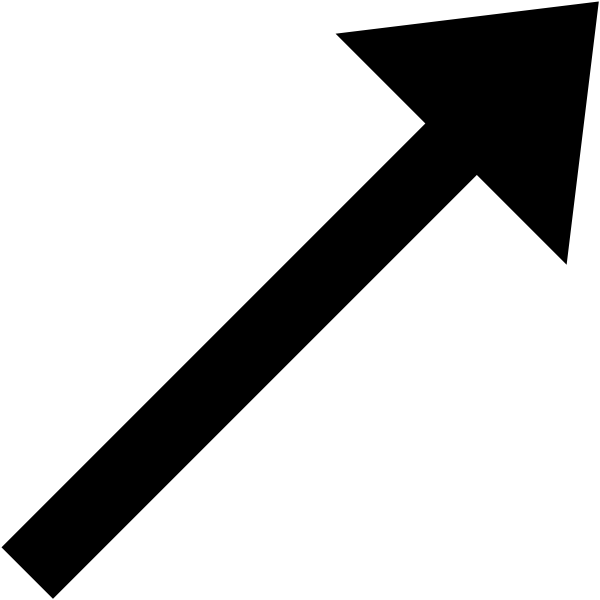
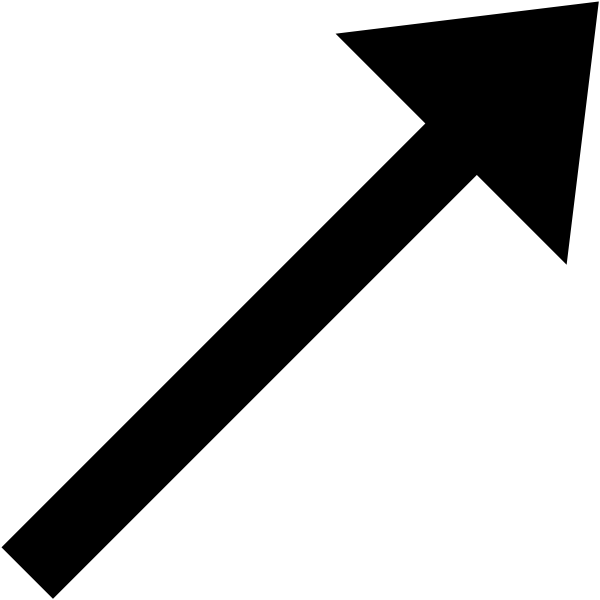
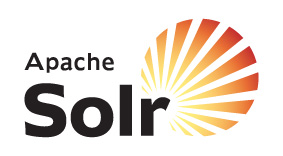
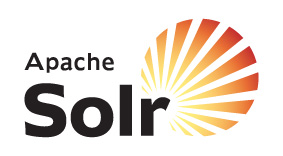
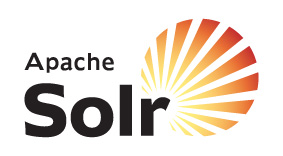
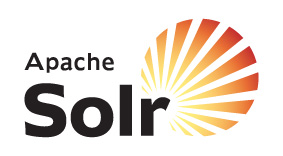
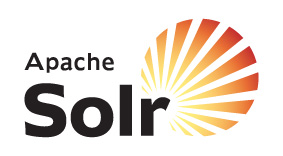
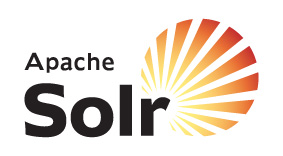
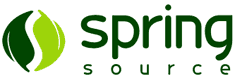
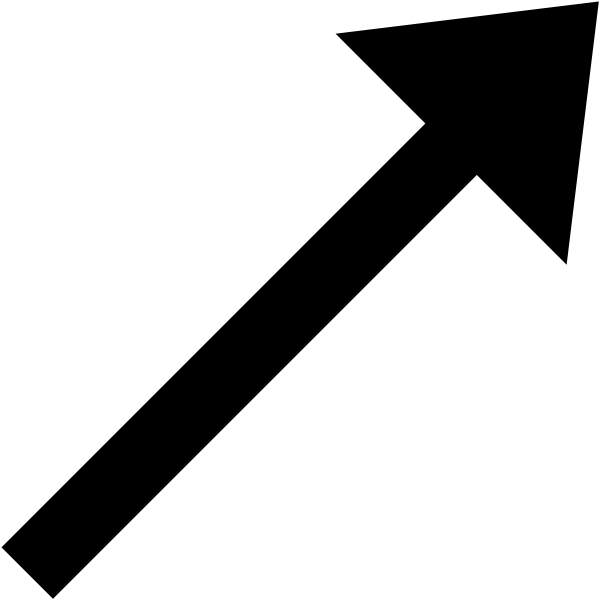
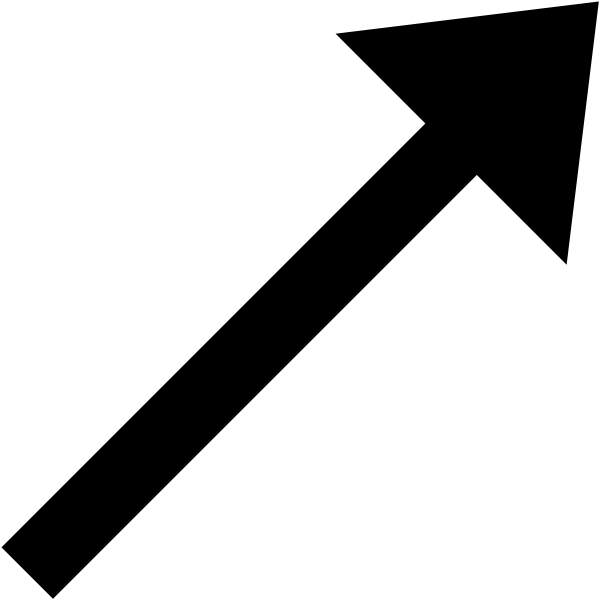
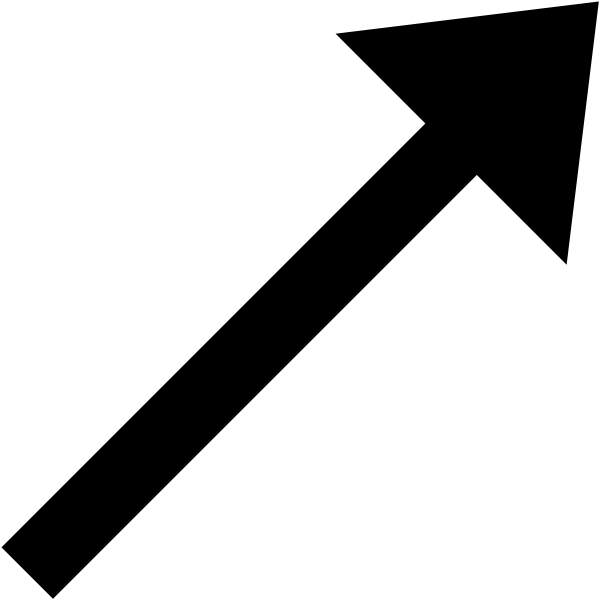
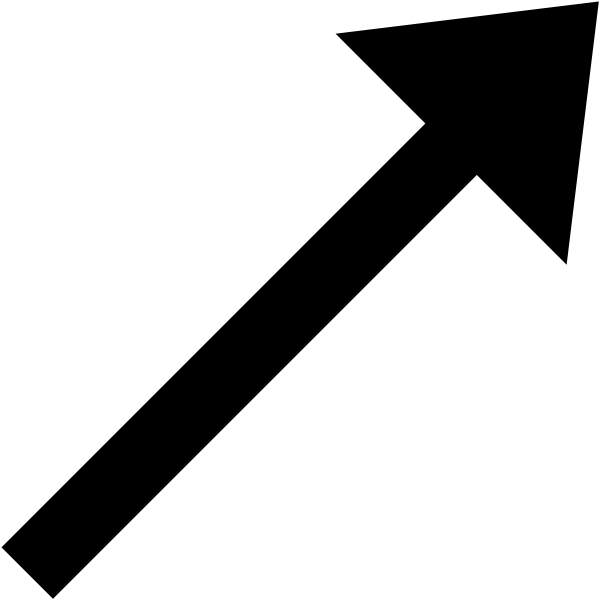
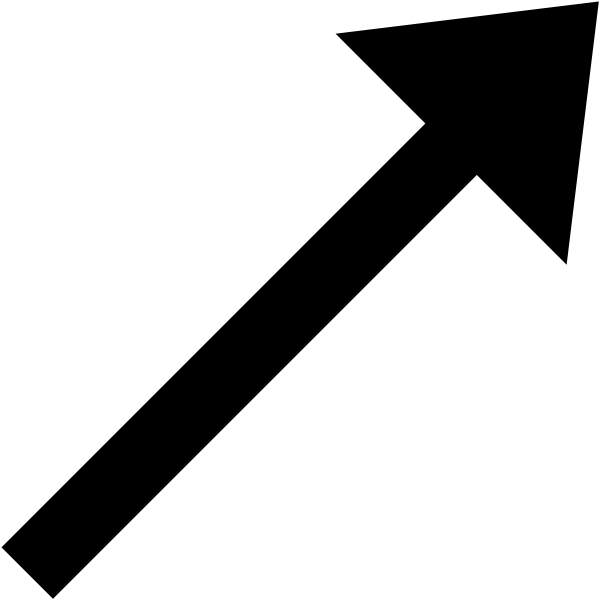
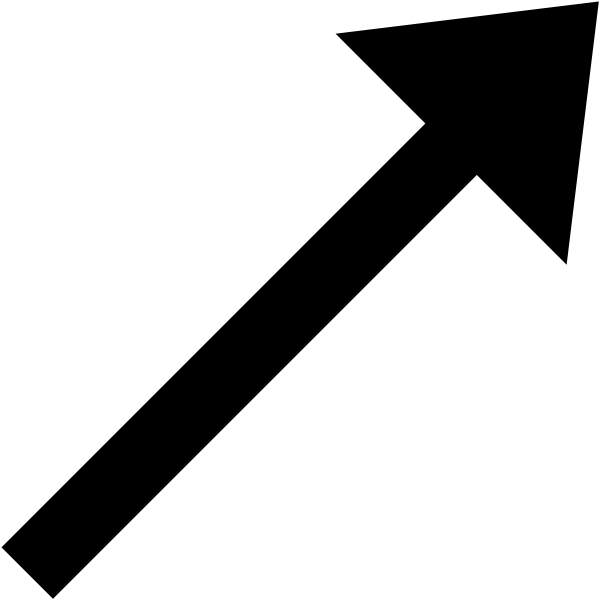
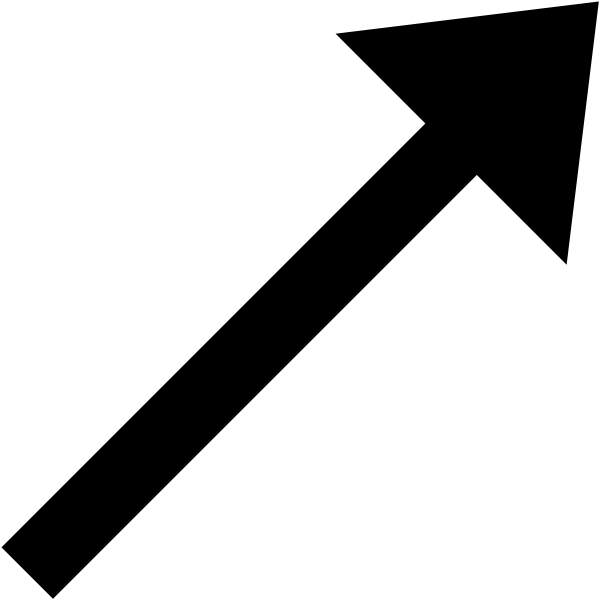
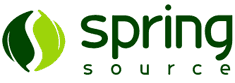
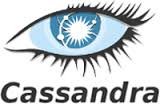
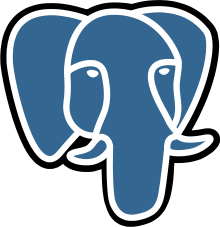
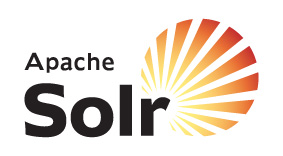
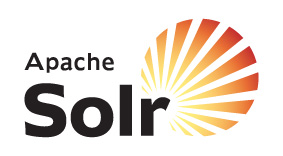
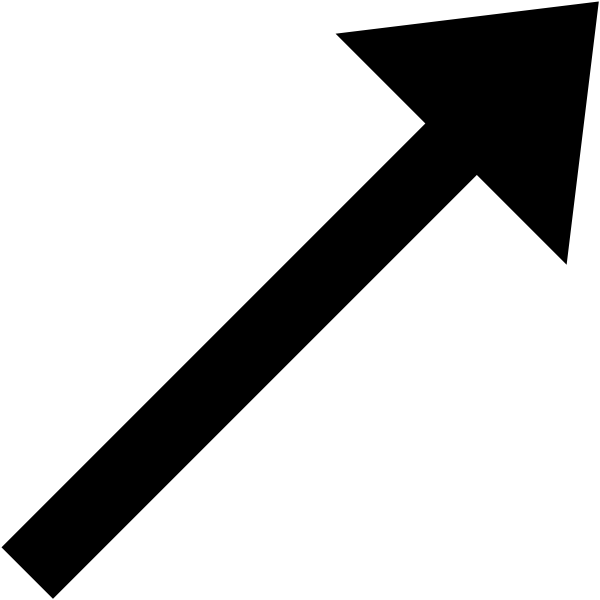
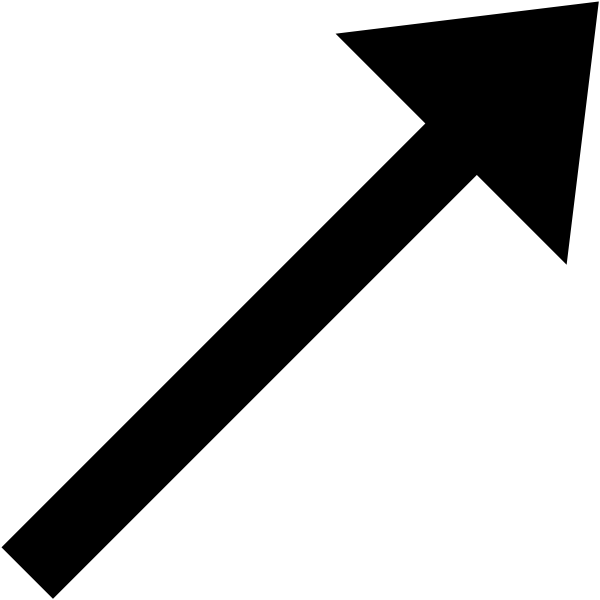
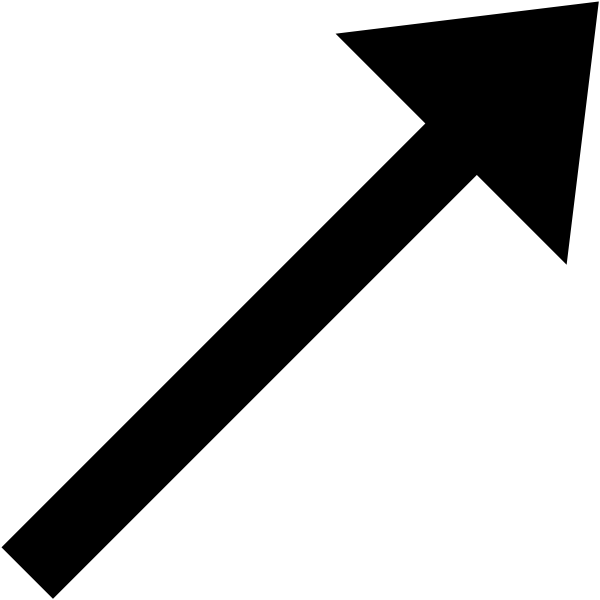
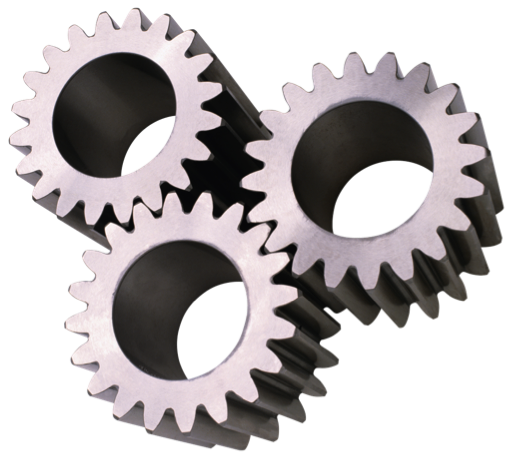
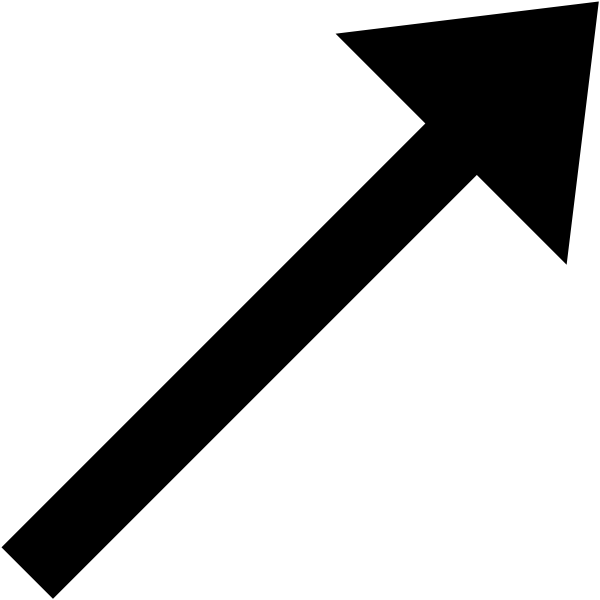
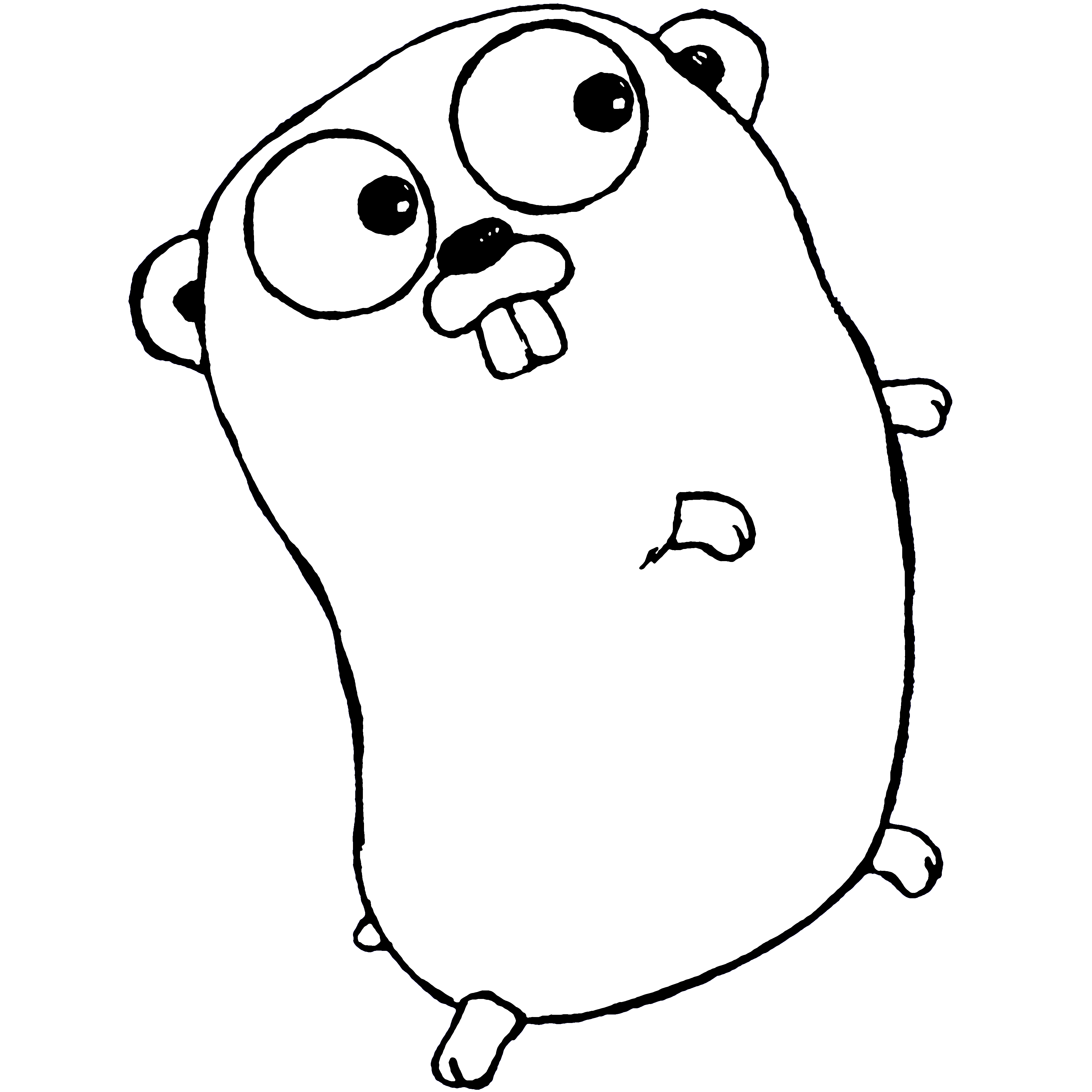
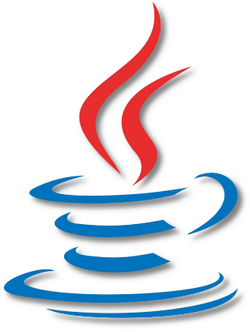
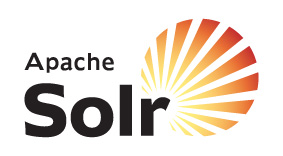
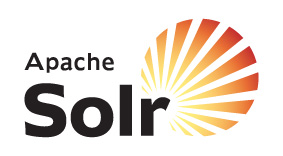
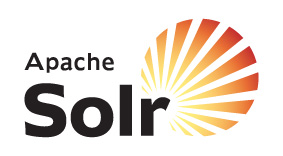
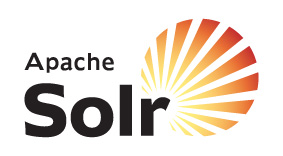
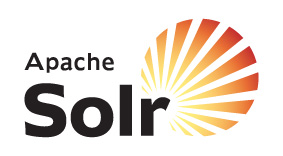
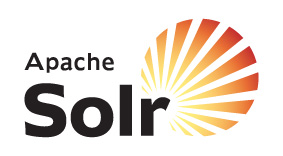
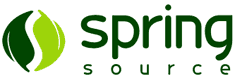
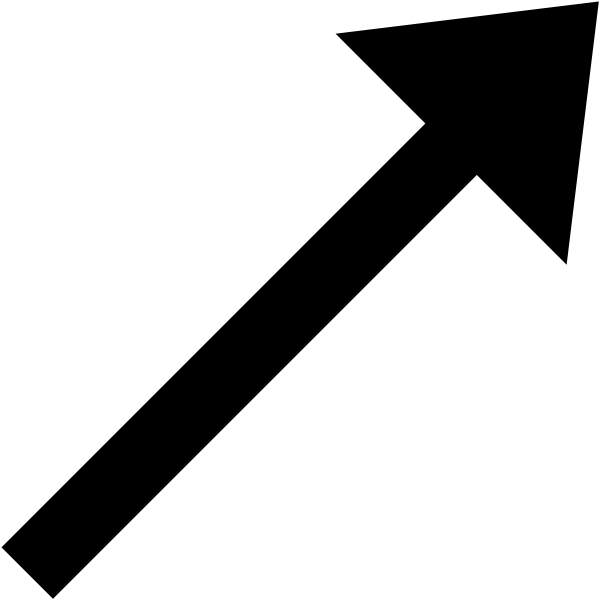
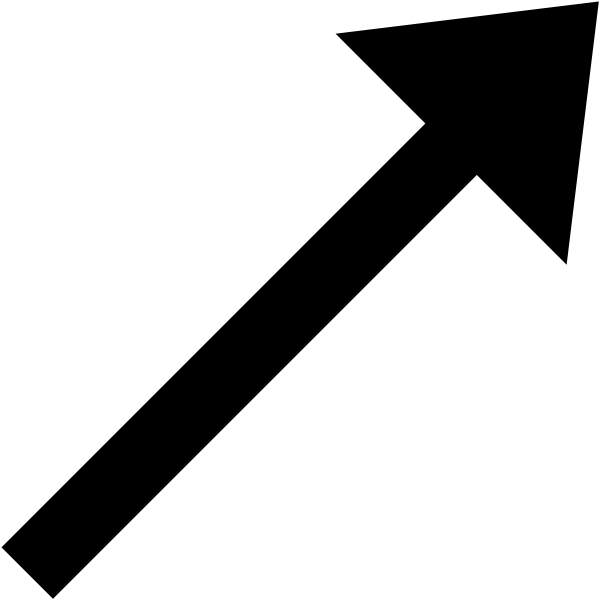
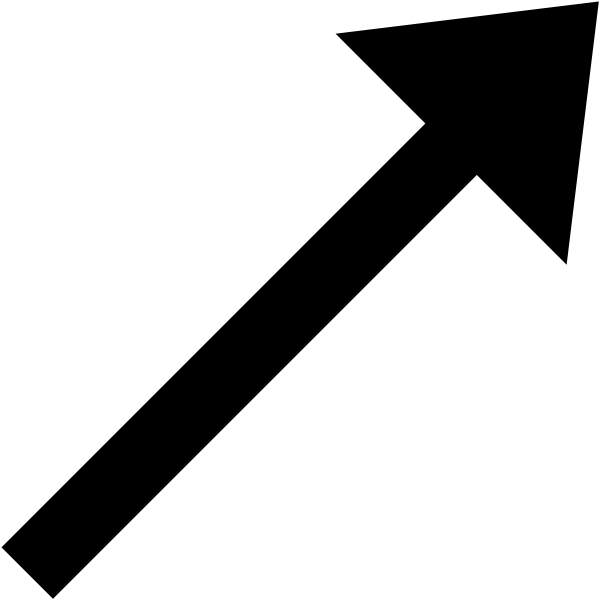
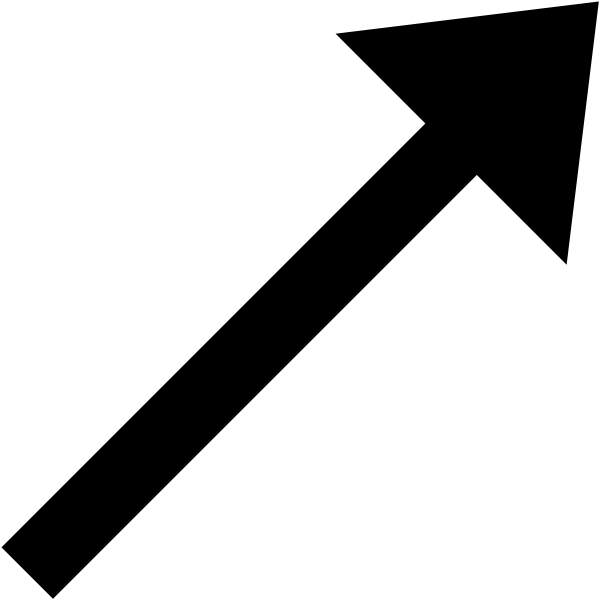
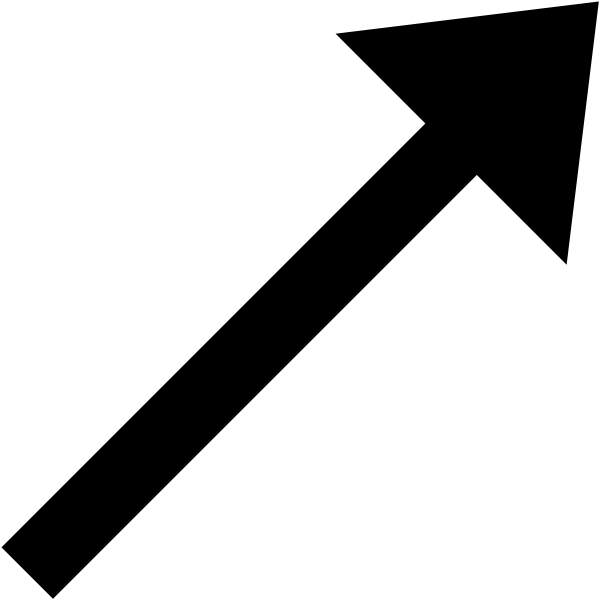
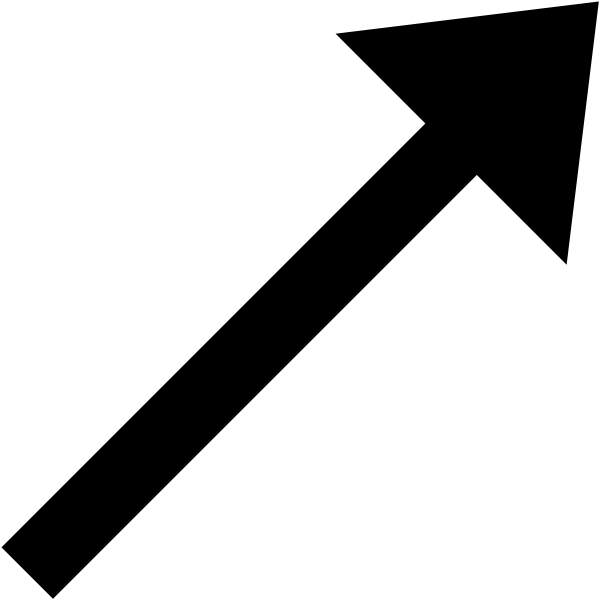
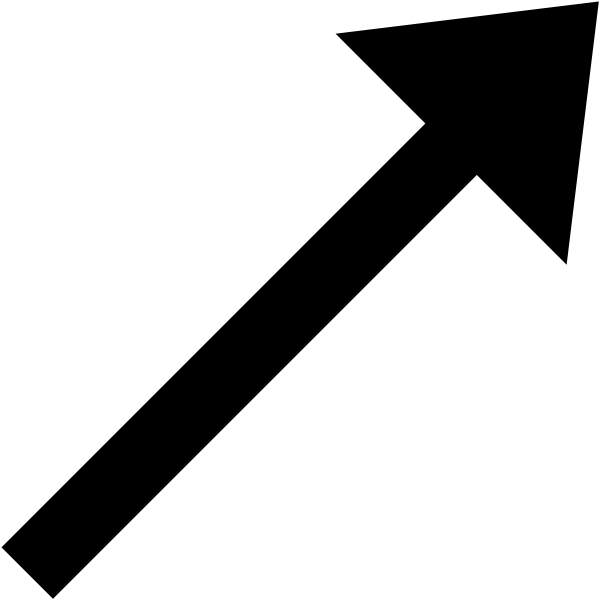
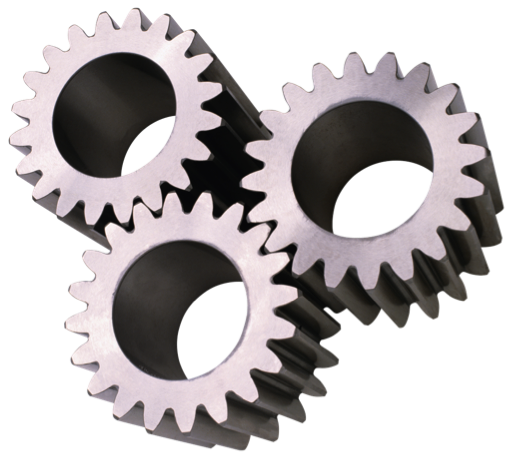
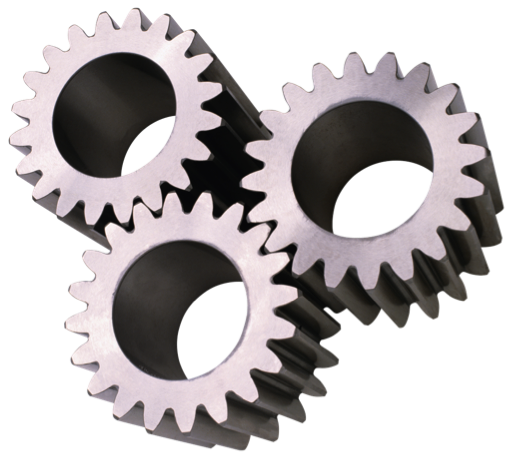
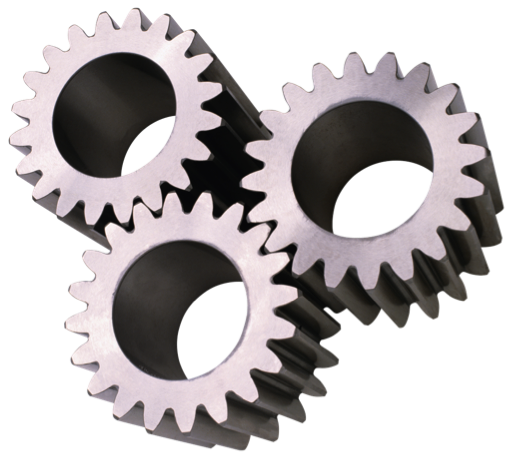
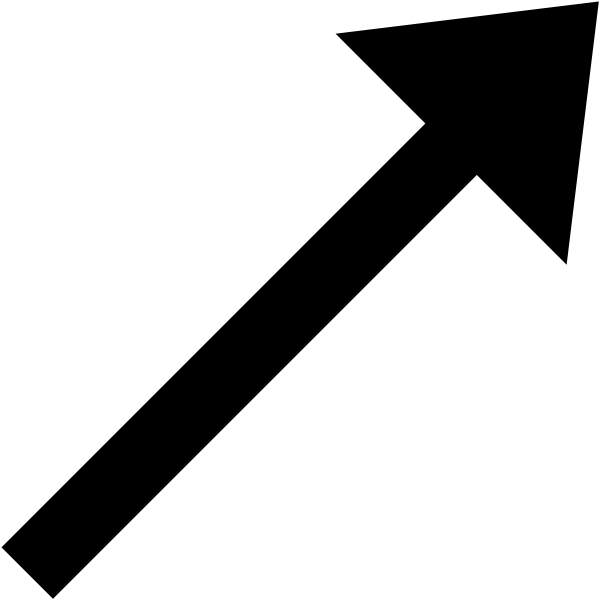
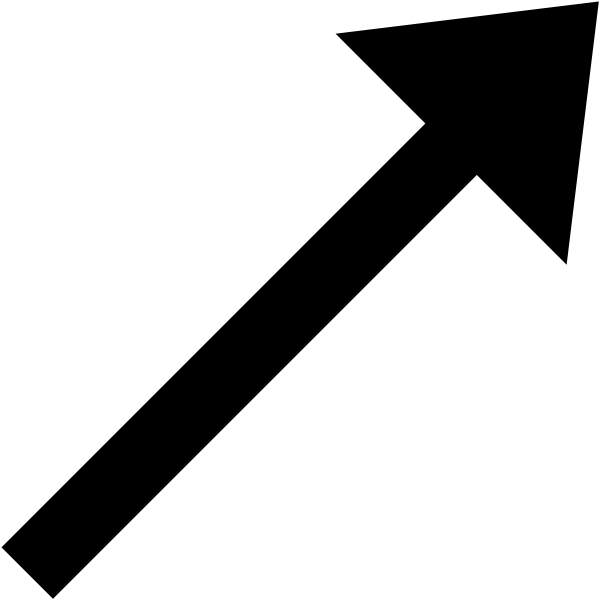
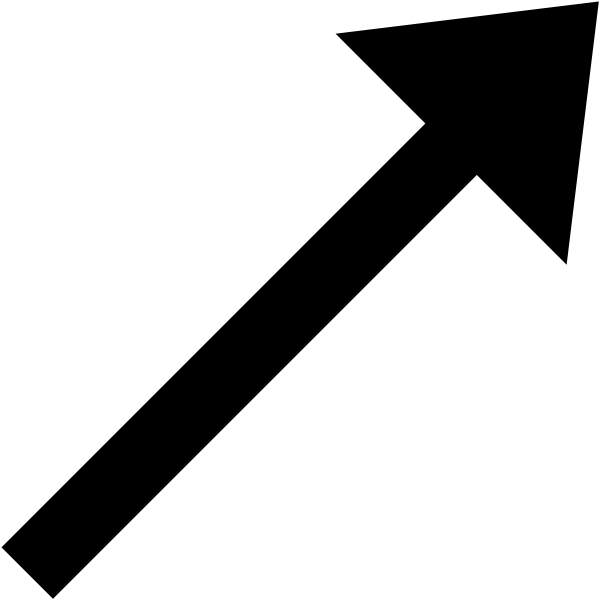
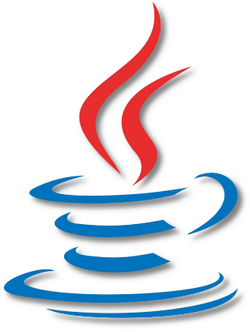
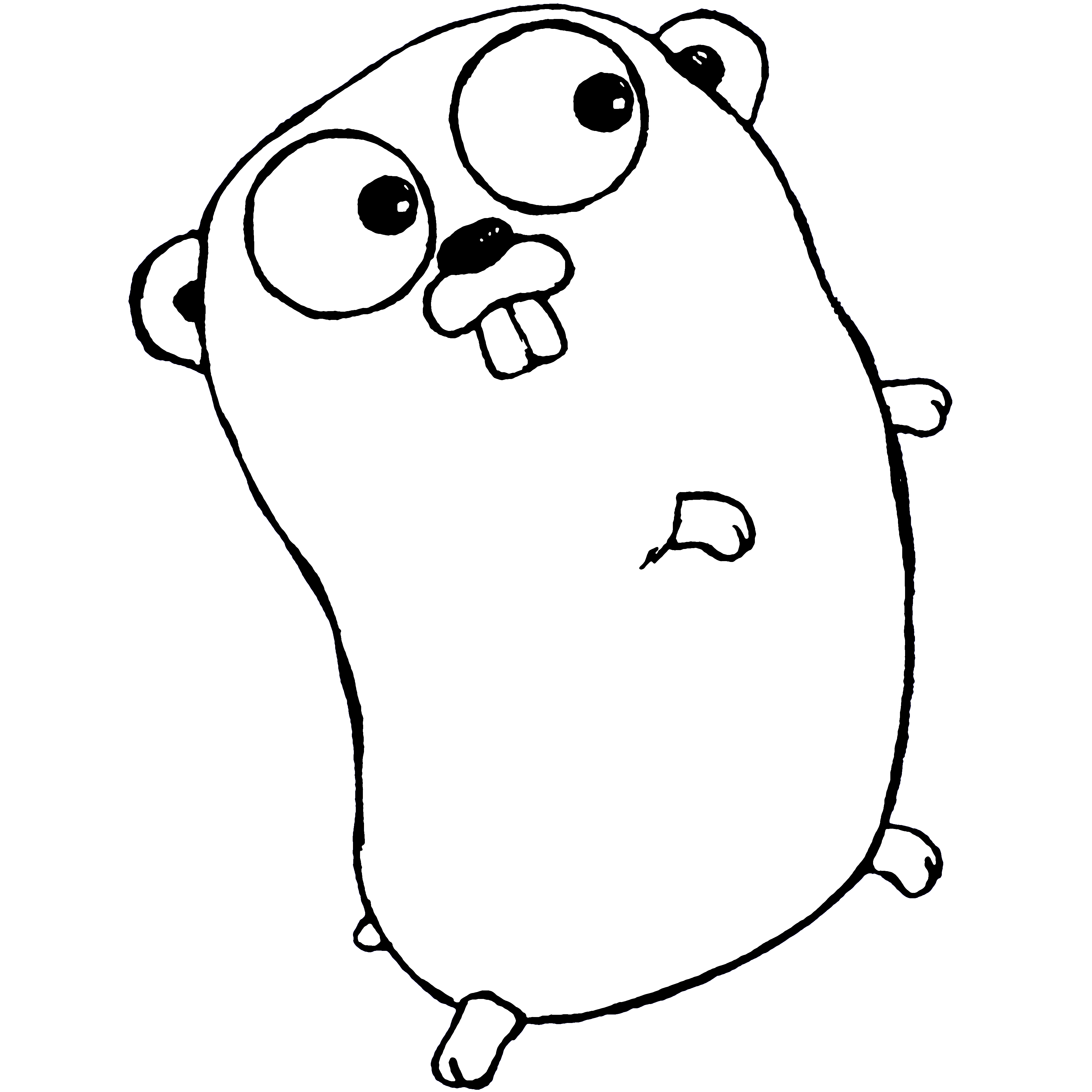
- We're Java Developers
- We have a similar implementation packaged into all of our sites
- We have the infrastructure in place to deploy java services
- It compiles easily and quickly into a command line tool
- It's standard libraries come with lots of webby goodness
- It mixes interesting programming paradigms (Functional + OO)
- Lot's of developers are talking about it! (and we want in)
http.Handle("/foo", fooHandler) http.HandleFunc("/bar", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, %q", html.EscapeString(r.URL.Path)) }) log.Fatal(http.ListenAndServe(":8080", nil))
s := &http.Server{ Addr: ":8080", Handler: myHandler, ReadTimeout: 10 * time.Second, WriteTimeout: 10 * time.Second, MaxHeaderBytes: 1 << 20, } log.Fatal(s.ListenAndServe())
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class Main {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
int[] vals = new int[]{7, 2, 8, -9, 4, 0};
int[] first = Arrays.copyOfRange(vals, 0, (int)Math.floor(vals.length/2));
int[] second = Arrays.copyOfRange(vals, (int)Math.floor(vals.length/2), vals.length);
ArrayList<Integer> results = new ArrayList<Integer>(2);
executor.execute(new Sum(first, results));
executor.execute(new Sum(second, results));
executor.shutdown();
try {
if (executor.awaitTermination(2l, TimeUnit.MINUTES)) {
System.out.println(String.format("%d, %d, %d", new Integer(results.get(0)), new Integer(results.get(1)), new Integer(results.get(0) + results.get(1))));
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
class Sum implements Runnable {
final int[] toSum;
final List<Integer> results;
public Sum(int[] toSum, List<Integer> results) {
this.toSum = toSum;
this.results = results;
}
@Override
public void run() {
int accum = 0;
for(int i:toSum) {
accum += i;
}
results.add(new Integer(accum));
}
}
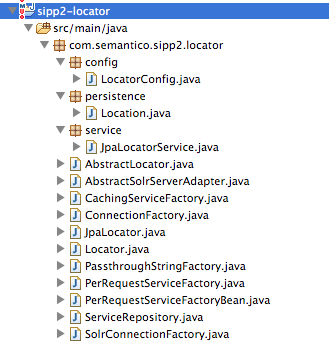
471 lines of code
424 lines of code
298 lines of code
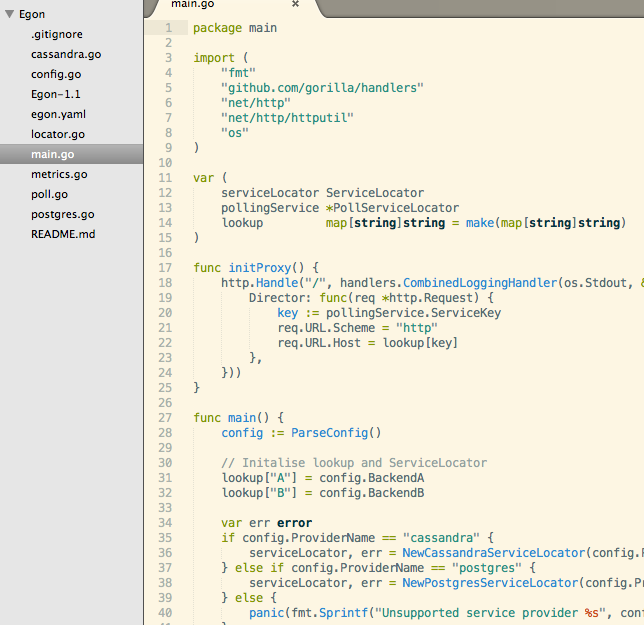
- Works with both Cassandra
- Has scope to work with other
- Configurable using a YAML file.
- Implements a http handler to serve
load balancers.
- Operates using a polling service,
either a default or the last successful
request to the service provider.
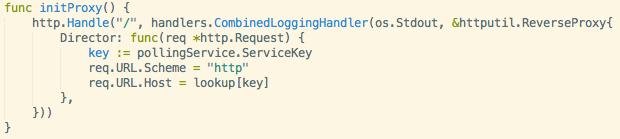

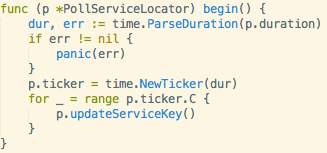

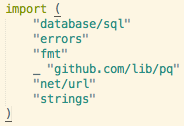



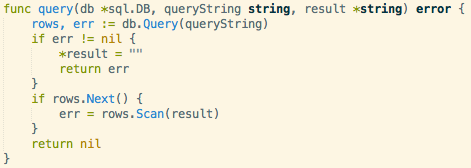
- 3 days to develop
- Fun to write
- Easy to understand compile time errors
- I had to manage my pointers
- I've run out of projects to write in Golang